Welcome to my BIGGEST GUIDE YET!
Iâve looked far and wide into the deepest depths of the forums and havenât found a reliable way to get every playerâs position and store it, and no way to find the distance between 2 players. UNTIL NOW! I have been working on this system for a while now. Bug fixing, optimizing, and Iâve finally gotten it to work. This will work for however many players you have in your game and doesnât require any manual input from the player (except them needing to move around ofc lol)! Letâs get to work!
What we'll be making
The overlay in the top left shows the playersâ position. The one in the bottom left shows the name, x, y, and distance of the closest player to you. (Disclaimer: this video is of the old version. The new version is a lot faster.)
Materials
This list is sorted first by the greatest number of devices to the least, then by how much memory they use.
Property Ă14 (140 memory)
Trigger Ă6 (3,240 memory)
Game Overlay Ă2 (1,350 memory)
Relay Ă2 (40 memory)
Speed Modifier Ă2 (20 memory)
Player Coordinates Ă1 (4,000 memory)
Lifecycle Ă1 (50 memory)
Counter Ă1 (25 memory)
Friends: 3 recommended, but good luck getting any
Total memory: 8,865
Now that you have everything you need, letâs get to it!
System setup
To make this system, we need every playerâs data to be the same length as everyone elseâs to prevent bugs. But usually, every player in the game has different names that are different lengths. To make the system work properly, everyoneâs name needs to be the same length. So, how do we do that? Well, the longest length that a player can set their name to is 20, so letâs make a system that does exactly that!
Letâs build it!
First, grab a lifecycle and set it up like this:
But if we just leave it like this, then the system will only work for the game host. So, we need to add a relay for the other players.
We broadcast on âGame start relayâ that way if thereâs ever another point where we need to make a system similar to this (when we want not only the game host to have the event happen for) then we can reuse this same lifecycle and relay for as many times as we need!
Now to make the actual system. Start by grabbing a Speed Modifier and set it up like this:
We need to do this that way the player canât move before we set their name, otherwise the system will break. Setting the playerâs speed to 0 will fix that.
Now, we can finally set the playerâs name! Get a property with these settings:
Now letâs make a trigger loop that will set the property for us.
Heads up!
All of the triggers in this guide have these settings enabled.
Have it trigger when receiving on this broadcast:
For more info on what this is and how this works, read this amazing guide by Blueboat!
Breaking the recursion cap | TUTORIAL | Difficulty: - Community Made Guides - Gimkit Creative
Yay first link!
Now make a new code block and make it like this:
Congratulations, youâve just made a trigger loop! This will set the âMy nameâ property until it has a length of 20!
Special character
For this system, I used a special character that appears invisible on most devices. This usually includes mobile devices, and macs, but I do not yet know whether or not it appears invisible on Chromebook. Iâm on windows, so for me, it looks like a little box, but since most people play Gimkit on a school laptop, this should work very well! Idk why it appears invisible in my pictures and not on my PC, but if you look at the GIF in the showcase, you should be able to see the boxes in the overlays.
Here is the character that I used. You should be able to copy-paste it into the text block in the photo that appears to be empty:
If youâre unable to do it here, then you can just look up âInvisible textâ in your browser and it should take you to a link where youâll be able to copy it by pressing a button. If everything is blocked for you, then you can go to scratch.mit.edu, go to the search bar, type in %15, and the character should be there where youâll easily be able to copy-paste it. If that doesnât work, womp womp lol.
Tracking every player's coords
Now that weâve set up our name, itâs time to track every playerâs coords at the same time! But once again, we want the length of the playerâs data to be the same as everyone elseâs. This means that the length of the players coords has to be the same as everyone elseâs. The largest number our coords can be is 640 which has a length of 3, so we want our coords to have a length of 3, even if our actual coords are lower than 100.
Time to build!
Get 4 new properties called âxâ, âyâ, âx spaceâ, and ây spaceâ.
Then get the âPlayer Coordinatesâ device and make a block like this:
If the length is already 3, then weâll go straight to save the playerâs position, otherwise, weâll use a trigger until the length is equal to 3. We use 2 separate properties that way we can use number properties for math, and text properties for spacing.
Letâs code the trigger that sets our spacing first. Have it trigger on âFix numbersâ.
Make a new block like this:
How it works
We combine the length of our actual coords with the length of the text to check how long it is. If itâs not equal to 3, then weâll just add on the special character to the front of the spacing property. Then we check if both lengths are greater than or equal to 2. If it says itâs equal to 2, then that means itâs actually equal to 3 because we just updated it.
Now letâs code the other trigger. But first, add 2 new properties like this:
Now we can finally code the trigger. Make it trigger when receiving on âUpdate positionâ like this:
Make a new block with this code:
How it works
WOW this is a lot of code. What does this even do?
Well, what the code does is get information from a property, figure out which part of the information belongs to the player, and replace it with our new information. Basically, weâre getting the substring of the property that contains all of the player data from the start to the individual players info, then we insert our new info, and then get the substring from the end of our info to the end of every playerâs info. However, if we canât find the playerâs data, then we add it on to the end of the property that contains all of the player data.
Youâve now successfully added a way to track every playerâs coords. Letâs add an overlay that displays all of the playersâ info. Add an overlay and make a new block:
Now the playersâ names and positions should appear on the overlay!
Tracking the distance between us and other players
This was the hardest part for me to figure out. You have to somehow get numbers out of a text property? How do you do that? Allow me to show you! But first, letâs make some new properties.
Now get a counter and give it these settings:
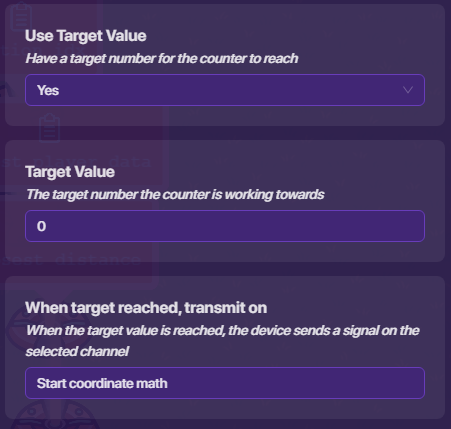
And get a relay:
Now get a trigger. Make it trigger when receiving on âCompare coordinatesâ:
Make a new block like this:
Now we need to make 3 more properties:
Now get a trigger that triggers on âText to numberâ:
Make a new block:
Make one last property called âClosest player dataâ:
And make one last trigger that triggers when receiving on âFunctionâ:
Make a block like this:
And now, you have completely made a system that will find the closest player to you!
How it works
Ima try to keep this short bc Iâm really tired of working on this lol.
The math behind setting the starting letter variable allows us to find the first letter in every 29 letters.
Special thanks to @TorontoBulls1 for showing me the system used to convert text into numbers. I have no idea how it works, but it does lol.
We use the Pythagorean theorem to find the distance between our coords and the player that weâre trackingâs coords, unless the player weâre tracking is ourselves.
We check if theyâre the closest player to us, then save everything to a property for later.
Then we restart the loop.
Now, letâs code an overlay that displays all of the information about the closest player.
Make sure the overlayâs position is in a different spot from the first one. I used bottom right.
Make a new block:
Now, get some friends into your game and start it! Does everything look correct? I sure hope so!
Disclaimer
This system might break if 2 people with the same name join, or if a player joins late. I tried to fix it for when a player joins late but it didnât work. However, you can disable late joining in your map settings:
Thanks for reading this guide! It took a very long time to make, but I think it was worth it!
What are some things this may be useful for? It could be used as a powerup in a battle royale to get the jump on your opponents! Or perhaps add a range to the distance, to make a honing missile using a damager, or do the same thing for a more realistic unaliving mechanic in among us games!
If youâre having any trouble building this, have any questions or found an optimization, drop a reply!
Canât wait to see what you guys come up with! Happy building!
âFulcrum-19
- AMAZING!!!
- Good!
- Ok.
- Bad.
- WORST GUIDE EVER!

- 0
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11